Overview : 카드번호를 넣고 5$을 결제해야 사용가능
- Endpoint : https://platform.openai.com/account/api-keys
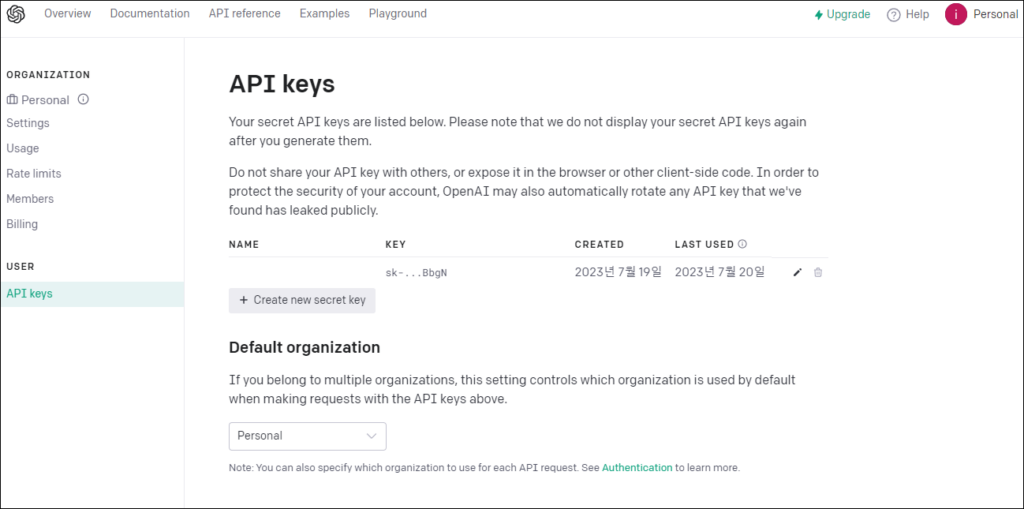
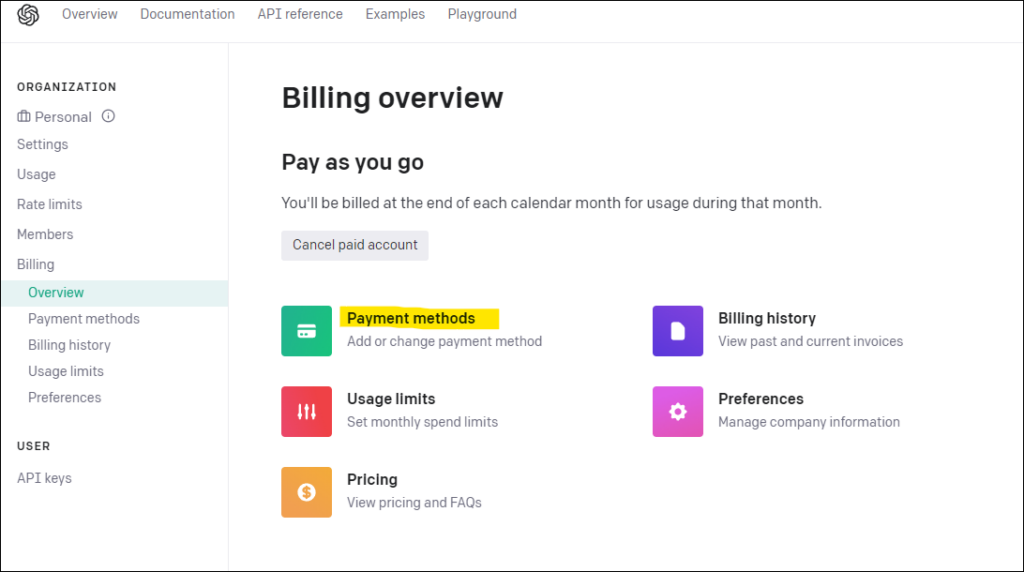
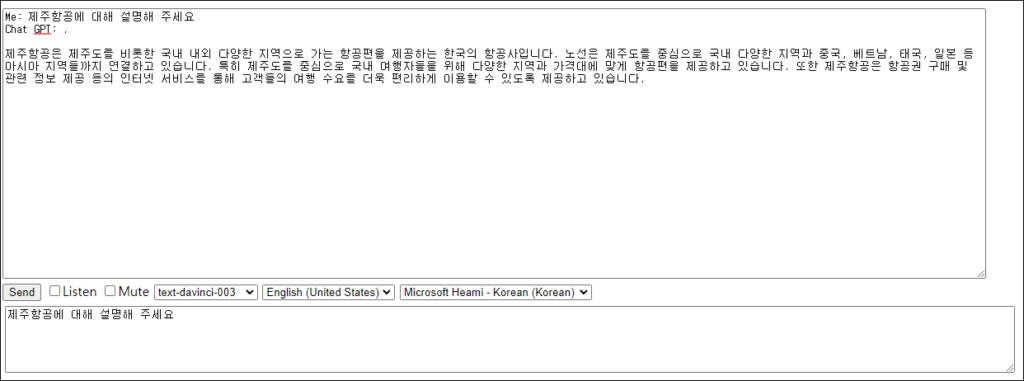
참조 : https://www.codeproject.com/Articles/5350454/Chat-GPT-in-JavaScript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
<!DOCTYPE html> <html> <head> <title>Chat GPT</title> <script src="ChatGPT.js?v=14"></script> </head> <body onload="OnLoad()"> <div id="idContainer"> <textarea id="txtOutput" rows="10" style="margin-top: 10px; width: 100%;" placeholder="Output"></textarea> <div> <button type="button" onclick="Send()" id="btnSend">Send</button> <label id="lblSpeak"><input id="chkSpeak" type="checkbox" onclick="SpeechToText()" />Listen</label> <label id="lblMute"><input id="chkMute" type="checkbox" onclick="Mute(this.checked)"/>Mute</label> <select id="selModel"> <option value="text-davinci-003">text-davinci-003</option> <option value="text-davinci-002">text-davinci-002</option> <option value="code-davinci-002">code-davinci-002</option> </select> <select id="selLang" onchange="ChangeLang(this)"> <option value="en-US">English (United States)</option> <option value="fr-FR">French (France)</option> <option value="ru-RU">Russian (Russia)</option> <option value="pt-BR">Portuguese (Brazil)</option> <option value="es-ES">Spanish (Spain)</option> <option value="de-DE">German (Germany)</option> <option value="it-IT">Italian (Italy)</option> <option value="pl-PL">Polish (Poland)</option> <option value="nl-NL">Dutch (Netherlands)</option> </select> <select id="selVoices"></select> </div> <textarea id="txtMsg" rows="5" wrap="soft" style="width: 98%; margin-left: 3px; margin-top: 6px" placeholder="Input Text"></textarea> <div id="idText"></div> </div> </body> </html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 |
var OPENAI_API_KEY = "<MY_API_KEY>"; var bTextToSpeechSupported = false; var bSpeechInProgress = false; var oSpeechRecognizer = null var oSpeechSynthesisUtterance = null; var oVoices = null; function OnLoad() { if ("webkitSpeechRecognition" in window) { } else { //speech to text not supported lblSpeak.style.display = "none"; } if ('speechSynthesis' in window) { bTextToSpeechSupported = true; speechSynthesis.onvoiceschanged = function () { oVoices = window.speechSynthesis.getVoices(); for (var i = 0; i < oVoices.length; i++) { selVoices[selVoices.length] = new Option(oVoices[i].name, i); } }; } } function ChangeLang(o) { if (oSpeechRecognizer) { oSpeechRecognizer.lang = selLang.value; //SpeechToText() } } function Send() { var sQuestion = txtMsg.value; if (sQuestion == "") { alert("Type in your question!"); txtMsg.focus(); return; } var oHttp = new XMLHttpRequest(); oHttp.open("POST", "https://api.openai.com/v1/completions"); oHttp.setRequestHeader("Accept", "application/json"); oHttp.setRequestHeader("Content-Type", "application/json"); oHttp.setRequestHeader("Authorization", "Bearer " + OPENAI_API_KEY) oHttp.onreadystatechange = function () { if (oHttp.readyState === 4) { //console.log(oHttp.status); var oJson = {} if (txtOutput.value != "") txtOutput.value += "\n"; try { oJson = JSON.parse(oHttp.responseText); } catch (ex) { txtOutput.value += "Error: " + ex.message } if (oJson.error && oJson.error.message) { txtOutput.value += "Error: " + oJson.error.message; } else if (oJson.choices && oJson.choices[0].text) { var s = oJson.choices[0].text; if (selLang.value != "en-US") { var a = s.split("?\n"); if (a.length == 2) { s = a[1]; } } if (s == "") s = "No response"; txtOutput.value += "Chat GPT: " + s; TextToSpeech(s); } } }; var sModel = selModel.value;// "text-davinci-003"; var iMaxTokens = 2048; var sUserId = "1"; var dTemperature = 0.5; var data = { model: sModel, prompt: sQuestion, max_tokens: iMaxTokens, user: sUserId, temperature: dTemperature, frequency_penalty: 0.0, //Number between -2.0 and 2.0 Positive value decrease the model's likelihood to repeat the same line verbatim. presence_penalty: 0.0, //Number between -2.0 and 2.0. Positive values increase the model's likelihood to talk about new topics. stop: ["#", ";"] //Up to 4 sequences where the API will stop generating further tokens. The returned text will not contain the stop sequence. } oHttp.send(JSON.stringify(data)); if (txtOutput.value != "") txtOutput.value += "\n"; txtOutput.value += "Me: " + sQuestion; txtMsg.value = ""; } function TextToSpeech(s) { if (bTextToSpeechSupported == false) return; if (chkMute.checked) return; oSpeechSynthesisUtterance = new SpeechSynthesisUtterance(); if (oVoices) { var sVoice = selVoices.value; if (sVoice != "") { oSpeechSynthesisUtterance.voice = oVoices[parseInt(sVoice)]; } } oSpeechSynthesisUtterance.onend = function () { //finished talking - can now listen if (oSpeechRecognizer && chkSpeak.checked) { oSpeechRecognizer.start(); } } if (oSpeechRecognizer && chkSpeak.checked) { //do not listen to yourself when talking oSpeechRecognizer.stop(); } oSpeechSynthesisUtterance.lang = selLang.value; oSpeechSynthesisUtterance.text = s; //Uncaught (in promise) Error: A listener indicated an asynchronous response by returning true, but the message channel closed window.speechSynthesis.speak(oSpeechSynthesisUtterance); } function Mute(b) { if (b) { selVoices.style.display = "none"; } else { selVoices.style.display = ""; } } function SpeechToText() { if (oSpeechRecognizer) { if (chkSpeak.checked) { oSpeechRecognizer.start(); } else { oSpeechRecognizer.stop(); } return; } oSpeechRecognizer = new webkitSpeechRecognition(); oSpeechRecognizer.continuous = true; oSpeechRecognizer.interimResults = true; oSpeechRecognizer.lang = selLang.value; oSpeechRecognizer.start(); oSpeechRecognizer.onresult = function (event) { var interimTranscripts = ""; for (var i = event.resultIndex; i < event.results.length; i++) { var transcript = event.results[i][0].transcript; if (event.results[i].isFinal) { txtMsg.value = transcript; Send(); } else { transcript.replace("\n", "<br>"); interimTranscripts += transcript; } var oDiv = document.getElementById("idText"); oDiv.innerHTML = '<span style="color: #999;">' + interimTranscripts + '</span>'; } }; oSpeechRecognizer.onerror = function (event) { }; } |