Overview : MVC + Login Sample

DB- employee
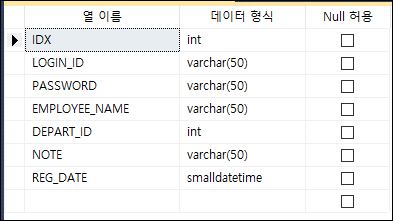
Employee Model
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
using System; using System.ComponentModel; using System.ComponentModel.DataAnnotations; using System.ComponentModel.DataAnnotations.Schema; namespace PRODUCT.Models { public class Employee { [Key] [Display(Name = "직원 일련번호")] public int IDX { get; set; } [Display(Name = "ID")] public string LOGIN_ID { get; set; } [Display(Name = "비밀번호")] public string PASSWORD { get; set; } = " "; [Display(Name = "성명")] public string EMPLOYEE_NAME { get; set; } = " "; [DefaultValue(true)] [Display(Name = "부서")] public int DEPART_ID { get; set; } = 1; [DefaultValue(true)] [Display(Name = "비고")] public string NOTE { get; set; } = " "; [DefaultValue(true)] [Display(Name = "등록일")] [DatabaseGenerated(DatabaseGeneratedOption.Identity)] [DataType(DataType.Date)] [DisplayFormat(DataFormatString = "{0:yyyy-MM-dd HH:mm}", ApplyFormatInEditMode = false)] public DateTime REGI_DATE { get; set; } } } |
Login View
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
@model PRODUCT.Models.Employee @{ ViewData["Title"] = "Index"; } <div class="container"> <div class="d-flex justify-content-center h-100"> <div class="card"> <div class="card-header"> <h3>Sign In</h3> <div class="d-flex justify-content-end social_icon"> <span><i class="fab fa-facebook-square"></i></span> <span><i class="fab fa-google-plus-square"></i></span> <span><i class="fab fa-twitter-square"></i></span> </div> </div> <div class="card-body"> @using (Html.BeginForm("Index", "Employees", FormMethod.Post)) { if (ViewBag.LoginStatus != null) { if (ViewBag.LoginStatus == 0) { <div class="alert alert-danger">Login 정보가 정확하지 않습니다.</div> } } <div class="input-group form-group"> <div class="input-group-prepend"> <span class="input-group-text"><i class="fas fa-user"></i></span> </div> @Html.TextBoxFor(model => model.LOGIN_ID, new { @class = "form-control", @placeholder = "ID" }) </div> <div class="input-group form-group"> <div class="input-group-prepend"> <span class="input-group-text"><i class="fas fa-key"></i></span> </div> @Html.PasswordFor(model => model.PASSWORD, new { @class = "form-control", @placeholder = "비밀번호" }) </div> <div class="row align-items-center remember"> <input type="checkbox">Remember Me </div> <div class="form-group"> <input type="submit" value="Login" class="btn float-right login_btn"> </div>} </div> <div class="card-footer"> <div class="d-flex justify-content-center links"> ID가 없으면 SD에 요청 바랍니다. </div> <div class="d-flex justify-content-center"> 비밀번호를 잊어버렸습니까? </div> </div> </div> </div> </div> |
Login Control
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
using System.Linq; using System.Threading.Tasks; using Microsoft.AspNetCore.Mvc; using Microsoft.EntityFrameworkCore; using PRODUCT.Data; using PRODUCT.Models; namespace REGRULE.Controllers { public class EmployeesController : Controller { private readonly VariableContext _context; public EmployeesController(VariableContext context) { _context = context; } // GET: Employees public IActionResult Index() { //return View(await _context.Employee.ToListAsync()); Employee _employee = new Employee(); return View(_employee); } //POST: Employees [HttpPost] public IActionResult Index(Employee employee) { var status = _context.Employee.Where(m => m.LOGIN_ID == employee.LOGIN_ID && m.PASSWORD == employee.PASSWORD).FirstOrDefault(); if (status == null) { ViewBag.LoginStatus = 0; } else { return RedirectToAction("index", "ProductDisplay"); } return View(employee); } } } |
Note : context.Database.EnsureCreated()는 컨텍스트에 대한 데이터베이스가 존재하는지 확인하는 새로운 EF 핵심 메서드입니다. 존재하는 경우 아무 조치도 취하지 않습니다. 존재하지 않는 경우 데이터베이스와 모든 스키마가 생성되고 이 컨텍스트에 대한 모델과 호환되는지 확인합니다.
DbInitializer.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
namespace PRODUCT.Data { public class DbInitalizer { public static void Initialize(VariableContext context) { context.Database.EnsureCreated(); } } } |
Note : OnModelCreating(DbModelbuilder modelbuilder) 메서드에서 관계를 지정해야 합니다. 따라서 모델이 처음 생성될 때 엔터티 간의 관계를 유지해야 합니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
using PRODUCT.Models; using Microsoft.EntityFrameworkCore; namespace PRODUCT.Data { public class VariableContext : DbContext { public VariableContext(DbContextOptions<VariableContext> options) : base(options) { } public DbSet<Variable_Data> Variable_Datas { get; set; } public DbSet<Employee> Employee { get; set; }//Employee protected override void OnModelCreating(ModelBuilder modeBuilder) { modeBuilder.Entity<Variable_Data>().ToTable("VARIABLE_DATA"); modeBuilder.Entity<Employee>().ToTable("Employee");//Employee } } } |