wkhtmltopdf
and wkhtmltoimage
are open source (LGPL) command line tools to render HTML into PDF
사이트 : http://wkhtmltopdf.org/index.html
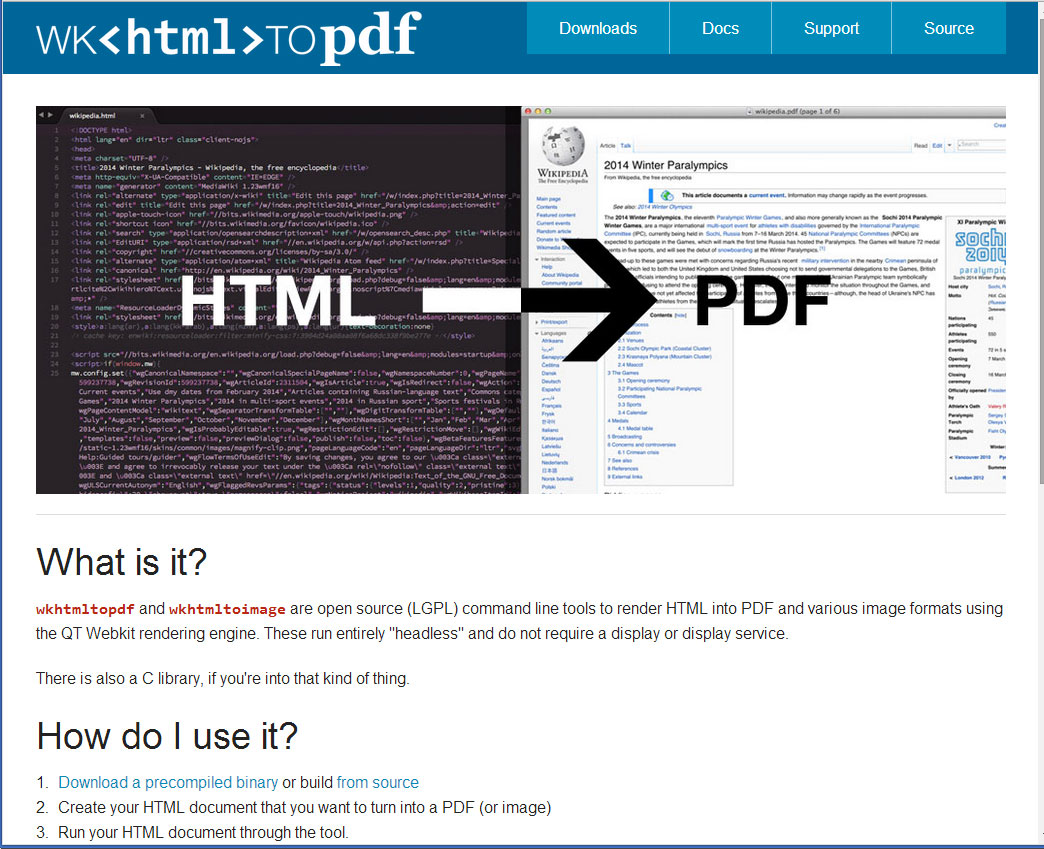
wkhtmltopdf 를 사용하여 html 을 pdf 로 만드는 c# 코드 샘플
우선, wkhtmltopdf
가 시스템에 설치되어 있어야 합니다. 여기에서 다운로드 및 설치할 수 있습니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
using System; using System.Diagnostics; class Program { static void Main(string[] args) { // HTML 파일 경로와 출력 PDF 파일 경로를 설정합니다. string htmlPath = @"C:\path\to\input.html"; string pdfPath = @"C:\path\to\output.pdf"; // wkhtmltopdf 실행 파일 경로를 설정합니다. string wkhtmltopdfPath = @"C:\path\to\wkhtmltopdf.exe"; // 명령줄 인수를 설정합니다. string arguments = $"\"{htmlPath}\" \"{pdfPath}\""; // ProcessStartInfo를 사용하여 프로세스를 구성합니다. ProcessStartInfo processStartInfo = new ProcessStartInfo { FileName = wkhtmltopdfPath, Arguments = arguments, UseShellExecute = false, RedirectStandardOutput = true, RedirectStandardError = true, CreateNoWindow = true }; try { // 프로세스를 시작합니다. using (Process process = Process.Start(processStartInfo)) { // 표준 출력 및 표준 오류 스트림을 읽습니다. string output = process.StandardOutput.ReadToEnd(); string error = process.StandardError.ReadToEnd(); // 프로세스가 완료될 때까지 대기합니다. process.WaitForExit(); // 프로세스 종료 코드를 확인합니다. if (process.ExitCode == 0) { Console.WriteLine("PDF 생성 성공!"); } else { Console.WriteLine("PDF 생성 실패:"); Console.WriteLine(error); } } } catch (Exception ex) { // 예외 처리 Console.WriteLine("예외 발생: " + ex.Message); } } } |
이 코드는 다음과 같이 동작합니다:
wkhtmltopdf
실행 파일, 입력 HTML 파일, 출력 PDF 파일의 경로를 설정합니다.ProcessStartInfo
를 사용하여wkhtmltopdf
명령을 실행하기 위한 프로세스를 구성합니다.- 프로세스를 시작하고 표준 출력 및 표준 오류 스트림을 읽습니다.
- 프로세스가 완료될 때까지 대기하고, 성공 여부를 확인합니다.
이 코드를 실행하기 전에, htmlPath
, pdfPath
, wkhtmltopdfPath
변수 값을 실제 경로로 변경해야 합니다. 또한, wkhtmltopdf
가 시스템 경로에 없을 경우 전체 경로를 지정해야 합니다.
이 코드를 통해 C#에서 wkhtmltopdf
를 사용하여 HTML 파일을 PDF로 변환할 수 있습니다.