메모리 캐시
속도 증가를 위해 디비 데이타를 미리 메모리에 가져오는 방식으로 변경해서 속도를 높이는 방식
장정 Pros
- Database 호출이 줄어든다.
- 웹서비스 로드가 줄어든다.
- 원하는 데이타를 빨리 찾을수 있다.
단점 Cons
- 유지관리가 많이 든다.
- 소스가 길어지고 복잡해진다.
- 데이타 베이스와 싱크를 맞추기가 어렵다.
Type
- In-Momory Cache : 짧은시간 사용하기
- 영구 Cache : 긴시간 사용하기
- 분산 Cashe : 여러프로세서로 분산하기
설치
NuGet 패캐지 관리에서 설치 및 참조 추가 확인 / 현재 8.0.0
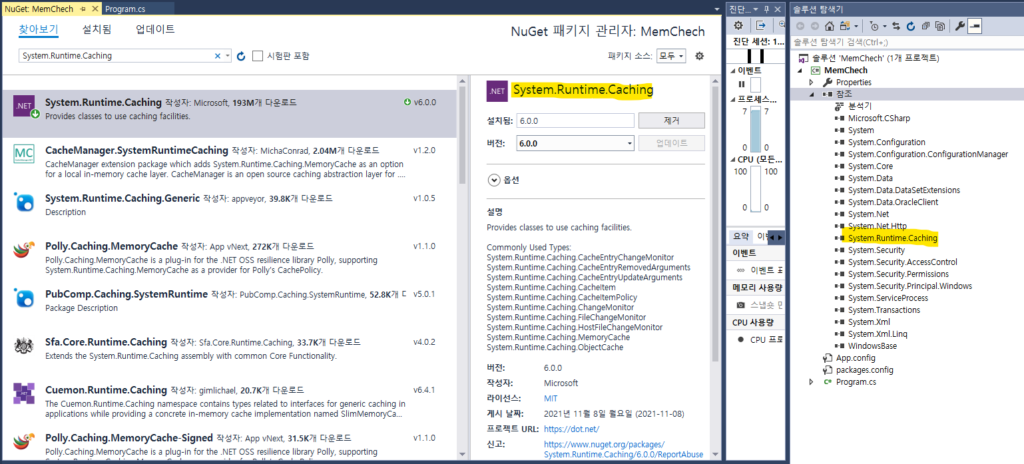
Sample 1
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
using System; using System.Data; using System.Data.SqlClient; using System.Runtime.Caching; namespace MemChech { class Program { static MemoryCache cache = new MemoryCache("DEV"); static void Main(string[] args) { var cacheItemPolicy = new CacheItemPolicy { AbsoluteExpiration = DateTimeOffset.Now.AddSeconds(60.0) }; var cacheItem = new CacheItem("fullName", "Hong Gildong"); var result = cache.Add(cacheItem, cacheItemPolicy); result = cache.Add("fullName02", "Hong Gildong02", cacheItemPolicy); result = cache.Add("fullName03", "Hong Gildong03", DateTimeOffset.Now.AddMinutes(10)); cache.Set(cacheItem, cacheItemPolicy); cache.Set("fullName04", "Hong Gildong04", cacheItemPolicy); var isExists = cache.Contains("fullName"); var value = cache.Get("fullName"); var getresult = cache.GetCacheItem("fullName"); var results = cache.GetValues(new string[] { "fullName", "fullName02", "fullName03", "fullName04" }); foreach (var item in results) { Console.WriteLine($"{item.Key} : {item.Value}"); } var removeresult = cache.Remove("fullName"); Console.WriteLine("fullName {0}", removeresult); var countresult = cache.GetCount(); Console.WriteLine(countresult); ///////////////////////////////////////// cache.Add(new CacheItem("firstName", "Jaimin02"), cacheItemPolicy); cache.Add(new CacheItem("lastName", "Shethiya"), cacheItemPolicy); results = cache.GetValues(new string[] { "firstName", "lastName", "fullName" }); foreach (var item in results) { Console.WriteLine($"{item.Key} : {item.Value}"); } countresult = cache.GetCount(); Console.WriteLine(countresult); Console.ReadLine(); } } } |
1 2 3 4 5 6 7 8 9 10 11 |
fullName : Hong Gildong fullName02 : Hong Gildong02 fullName03 : Hong Gildong03 fullName04 : Hong Gildong04 fullName Hong Gildong 3 firstName : Jaimin02 lastName : Shethiya 5 |
Sample 2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
private void Page_Load(object sender, System.EventArgs e) { DataSet ds=(DataSet)HttpContext.Current.Cache["dataset"]; if(ds==null) { HttpContext.Current.Cache["dataset"]= DAL.DataUtil.GetDataSet("server=peterlap;DataBase=Northwind;user id=sa;","Select * from cUstomers"); } ds=(DataSet)HttpContext.Current.Cache["dataset"]; DataGrid1.DataSource=ds.Tables[0]; DataGrid1.DataBind(); DataSet ds2=(DataSet)HttpContext.Current.Cache["dataset2"]; if(ds2==null) { HttpContext.Current.Cache["dataset2"]= DAL.DataUtil.GetDataSet("server=peterlap;DataBase=Northwind;user id=sa;","Select * from employees"); } ds2=(DataSet)HttpContext.Current.Cache["dataset2"]; DataGrid2.DataSource=ds.Tables[0]; DataGrid2.DataBind(); } public static DataSet GetDataSet(string strConnString, string strSQL) { SqlConnection cn = new SqlConnection(strConnString); cn.Open(); SqlCommand cmd =new SqlCommand(strSQL, cn); SqlDataAdapter da = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); da.Fill(ds); cn.Close(); return ds; } |